Your first face detection program with OpenCV
According to Grand View Research, the global computer vision market size was valued at USD 11.32 billion in 2020 and is expected to expand at a compound annual growth rate (CAGR) of 7.3% from 2021 to 2028.
The COVID-19 has boosted the computer vision market. Computer vision, a subset of AI, has enjoyed recent success in handling complex healthcare tasks related to controlling the spread of disease.
Currently, computer vision is used for:
- face mask detection
- automatic fever screening
- touchless authentication, etc.
A common problem in Computer Vision is the face detection, which can of course, get solved by many approaches such as Machine Learning or Deep Learning. This time we will use one of the simplest technical approaches available to provide a quick start for those who begin to study computer vision.
Haar Cascades Classifiers
Introduced by Paul Viola and Michael Jones is a Machine Learning based approach, which means we have to provide labeled data. Initially we will need to train the classifier by providing a lot of positive and negative examples and finally extract the best features. The Haar-like features are used as they are digital images features used for objects recognition, but even that way that’s a lot of calculations, so they introduced the Cascade Classifiers, based on the fact that most of the area of a face image is not actually face area so it would be inefficient to apply all there features in a non-face area. Cascade Classifiers are grouped by stages and applied one by one so non-face images are detected in early stages with no need of extra processing.
In OpenCV
To create our own classifier OpenCV provides a trainer, but it also provides pre-trained classifiers as XML files stored in the haarcascades directory under your OpenCV installation path. For our project we will be using haarcascade_frontalface_default.xml
Hands on
Now that we are ready to start, so we need something to classify.
Mat image;
In Computer Vision terms an image is a multidimensional matrix, a cv::Mat object, which contains every pixel of the image and this pixel has 3 channels: Blue, Green and Red.
image = imread("data/img.jpg");
The simplest method to load an image is cv::imread, it needs the path to the image and the flags which by default is IMREAD_COLOR, it means the image will have the 3 color channels available, we could also use IMREAD_GRAYSCALE if needed, there are off course many more available you can find in the official documentation
Let’s create our classifier and load the training data.
CascadeClassifier classifier;
classifier.load("/usr/local/share/OpenCV/haarcascades/haarcascade_frontalface_default.xml");
Once achieved this point we proceed to create a container for the detections and start using our classifier
vector<cv::Rect> detected_objects; classifier.detectMultiScale( image, detected_objects );
Now we can check for the size of the container in order to iterate over it and display the results
for ( size_t i = 0; i < detected_objects.size(); i++ ){ rectangle(image, detected_objects[i], Scalar(0, 0, 255)); }
Here we use the cv::rectangle function to show the detection area, it takes as parameters a cv::Mat object where the rectangle will be painted, a cv::Rect object containing the dimensions of the rectangle and a cv::Scalar to describe the color in Blue-Green-Red (BGR). And finally our program will show the desired output.
imshow("result",image );
And we should get something like this
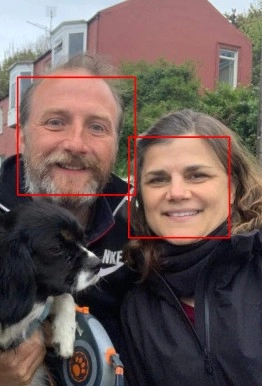
Conclusion
Face detection is the base for facial recognition, facial motion capture, photography, marketing, emotional inference lip reading and as further as your creativity allows you. This program’s accuracy can be improved by defining the scaleFactor, minNeighbors, minSize and maxSize parameters in the detectMultiScale function of our classifier, the first one refers to how much the image size is reduced at each image scale, a smaller number represents smaller step for downscaling, the second specifies how many “neighbors” every candidate should have, a bigger value represents less results with better quality and finally you can use the size parameters to calibrate you results in terms of how big or small faces you want to detect.
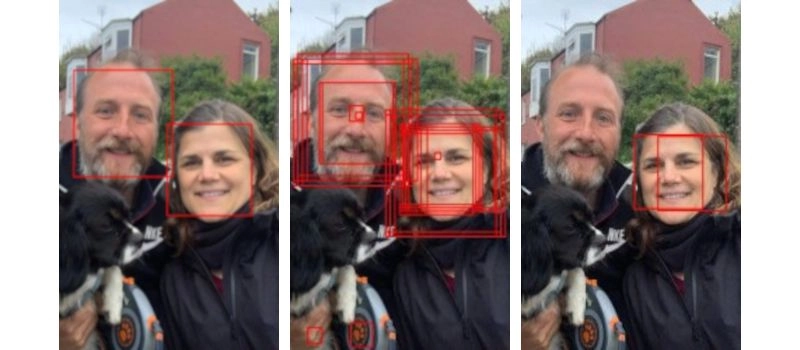
Try it on your own
OpenCV has many classifiers training data available in the haarcascades directory, like plate number detector, smile and more. This code is available on GitHub for you as a template for you to start your own project. Happy hacking!